Building a Serverless API with AWS API Gateway AWS Lambda and DynamoDB
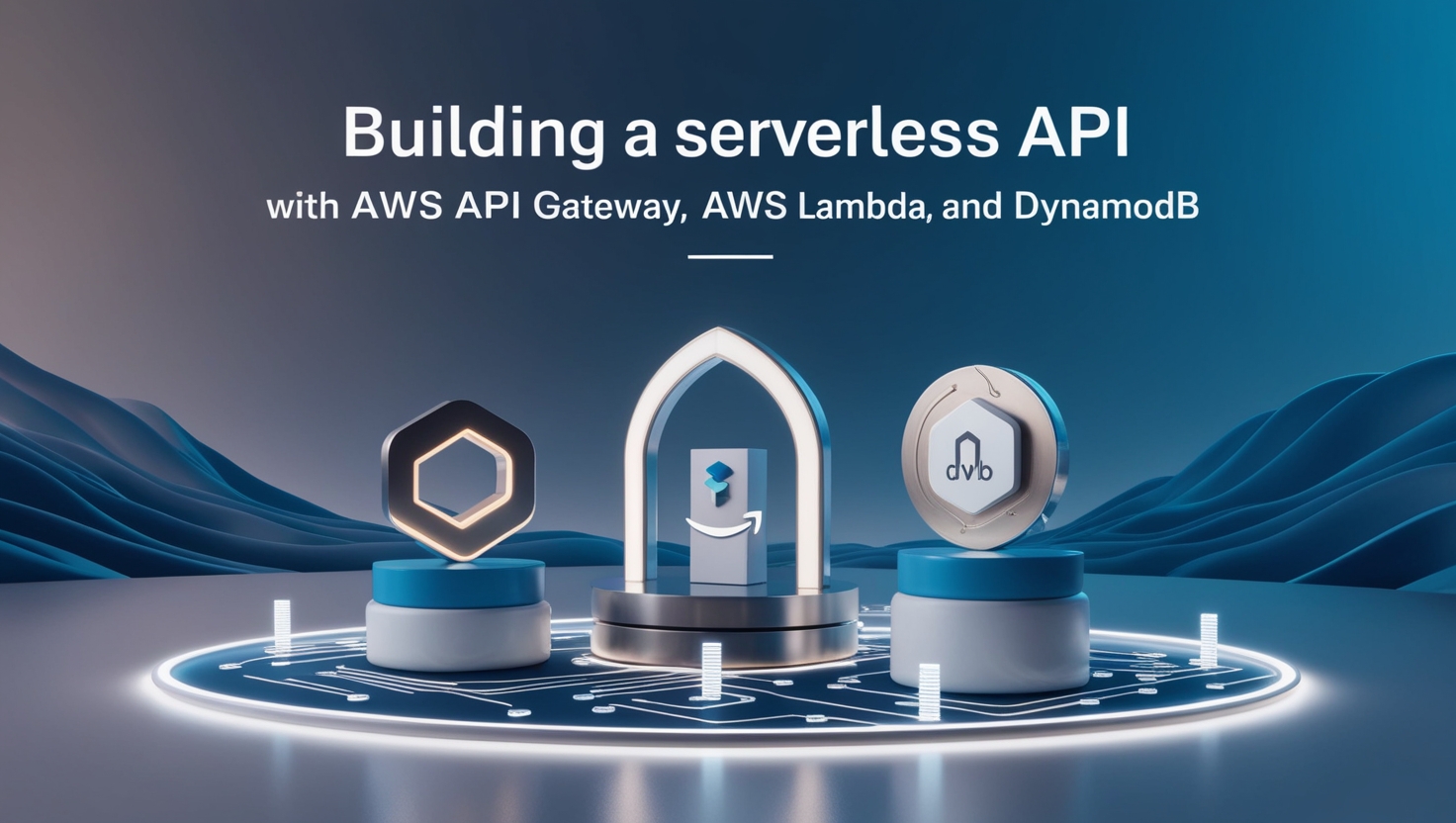
Serverless computing has revolutionized the way developers build and deploy applications by removing the need to manage server infrastructure. AWS offers powerful tools such as AWS API Gateway and AWS Lambda to create robust serverless APIs. This blog will guide you through the process of building a serverless API step by step.
What is a Serverless API?
A serverless API enables you to expose functionality over HTTP/S endpoints without provisioning or managing servers. AWS Lambda executes backend logic in response to API calls, while AWS API Gateway acts as a front-door for API traffic.
Why Use AWS API Gateway and AWS Lambda?
- Scalability: Automatically scales with traffic.
- Cost-Effectiveness: Pay only for what you use.
- Ease of Use: AWS provides a streamlined setup for API creation.
- Integration: Easily connects to other AWS services.
Overview
Here’s how the system will work:
- API Gateway will manage the HTTP requests and route them to the appropriate Lambda functions.
- Lambda Functions will handle the business logic:
- A POST function to create new records in DynamoDB.
- A GET function to fetch all records from DynamoDB.
- DynamoDB will store the application data.
This architecture eliminates the need for server management, allowing you to focus on your API logic.
Prerequisites
Before starting, ensure the following:
- An AWS account.
- AWS CLI installed and configured (aws configure).
- Basic knowledge of AWS Lambda, API Gateway, and DynamoDB.
Step 1: Create a DynamoDB Table
First, we’ll create a DynamoDB table to store our API data.
Run the following AWS CLI command to create a table named Users with a primary key userId:
aws dynamodb create-table \ –table-name Users \
–attribute-definitions AttributeName=userId,AttributeType=S \
–key-schema AttributeName=userId,KeyType=HASH \
–provisioned-throughput ReadCapacityUnits=5,WriteCapacityUnits=5
This will create a table with userId as the partition key.
Step 2: Write Lambda Functions
We’ll create two Lambda functions—one for handling GET requests and another for POST requests.
1. GET Lambda Function
This function retrieves all records from the Users table.
Save the following code as get_users.py:
|
2. POST Lambda Function
This function inserts a new record into the Users table.
Save the following code as post_users.py
|
Step 3: Deploy Lambda Functions
1. Create ZIP Packages
Compress the Lambda code into ZIP files:
zip get_users.zip get_users.py zip post_users.zip post_users.py
2. Deploy Lambda Functions
Deploy the functions using the AWS CLI:
|
Step 4: Configure API Gateway
1. Create an API
Create a new REST API:
|
Note the restApiId from the output.
2. Create Resources
Add a resource /users:
|
Note the resourceId from the output.
3. Add Methods
- POST Method:
|
Integrate the POST method with the PostUsersFunction Lambda:
|
- GET Method:
|
Integrate the GET method with the GetUsersFunction Lambda:
|
Step 5: Deploy the API
Deploy the API to a stage:
|
Step 6: Grant API Gateway Permissions
Grant API Gateway permission to invoke the Lambda functions:
|
Step 7: Test the API
Use curl or Postman to test the API:
- POST Request:
|
|
- GET Request:
|
Conclusion
In this tutorial, we built a serverless REST API using AWS Lambda, API Gateway, and DynamoDB. This architecture enables seamless scaling and minimal maintenance, making it perfect for cloud-native applications. You can extend this API by adding authentication, custom domains, or additional endpoints. Serverless is the future—dive in and explore!